A Complete Guide to Event and Listener in Laravel
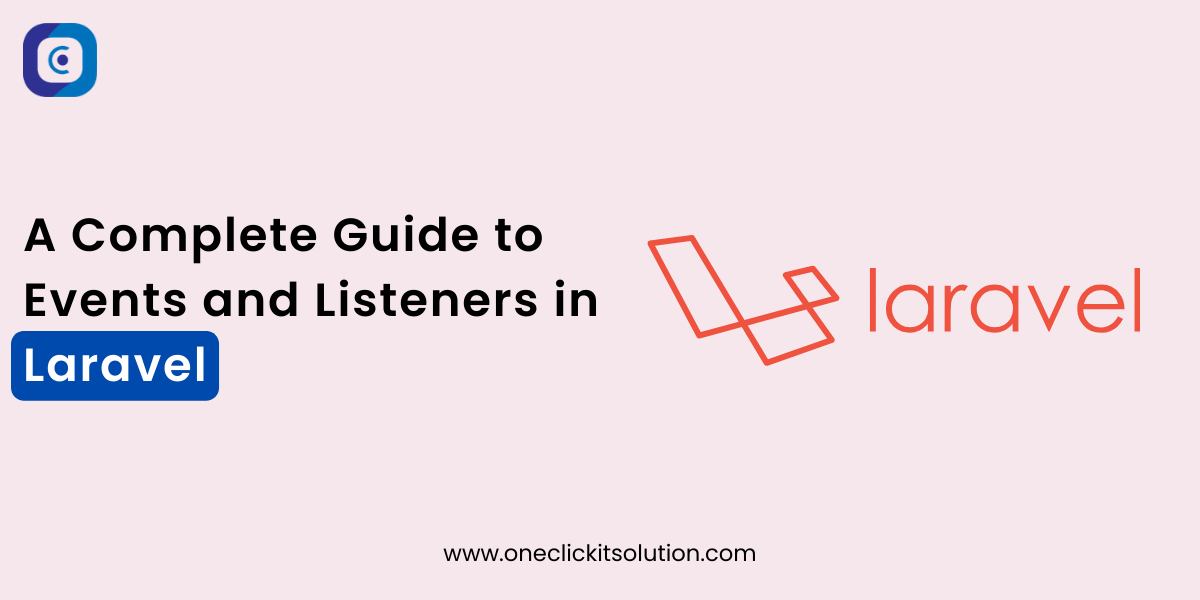
In the world of Laravel development, handling event and listener is a crucial part of building scalable and maintainable applications. The event-driven architecture allows Laravel developers to break down complex logic into smaller, more manageable components. In this blog, we will explore what events and listeners are in Laravel, how they work, and how you can effectively implement them in your projects.
What Are Events and Listeners in Laravel?
In Laravel, events are actions or occurrences within your application that you want to handle in response to a certain action. A listener is a class that reacts to an event, often performing some task or logic when the event is triggered. Together, events and listeners provide a decoupled way to manage your application’s logic, making it easier to maintain and scale.
How Do Events and Listeners Work in Laravel?
Laravel’s event and listener system is based on the observer design pattern. When an event occurs, it “fires” and the corresponding listener reacts to that event. For example, when a new user registers on your platform, an event such as UserRegistered
might fire, triggering a listener to send a welcome email.
Here’s a brief breakdown of how they work:
- Event: A specific action that happens in your application (e.g., user login, payment processed).
- Listener: The code that listens for that event and executes a task when it’s triggered (e.g., sending an email, logging data).
Creating an Event and Listener in Laravel
Step 1: Create an Event
You can generate a new event using Artisan, Laravel’s command-line tool. For example:
php artisan make:event UserRegistered
This command creates a new event class in the app/Events
directory. In the class, you can define the properties and methods related to the event.
Step 2: Create a Listener
To create a listener that reacts to the UserRegistered
event, run the following command:
php artisan make:listener SendWelcomeEmail --event=UserRegistered
This creates a listener class in the app/Listeners
directory. The listener class contains the logic that should run when the event is triggered.
Step 3: Register Events and Listeners
You can register events and listeners in the EventServiceProvider
located in the app/Providers
directory. In the boot()
method, you’ll map your events to their listeners:
php
protected $listen = [ 'App\Events\UserRegistered' => [ 'App\Listeners\SendWelcomeEmail', ], ];
This tells Laravel to trigger the SendWelcomeEmail
listener whenever the UserRegistered
event occurs.
Step 4: Trigger the Event
To trigger the event, simply fire it from anywhere in your application:
php
event(new UserRegistered($user));
Common Use Cases for Events and Listeners
- Sending Emails: Automatically send a confirmation or welcome email when a user registers.
- Logging Activities: Log user actions like login, registration, or profile updates.
- Real-Time Notifications: Send notifications to users when certain actions happen (e.g., a new comment on their post).
- Audit Trail: Maintain a record of certain actions for auditing purposes.
Benefits of Using Events and Listeners in Laravel
- Separation of Concerns: By decoupling logic, events and listeners make your code more modular and easier to maintain.
- Scalability: As your application grows, adding new events and listeners is easier than modifying existing logic.
- Reusability: You can reuse the same event in different parts of your application with different listeners.
- Improved Readability: Events and listeners make your code more readable by isolating logic into specific, self-contained classes.
Conclusion
Events and listeners are powerful tools that enable Laravel developers to write clean, maintainable, and scalable applications. By leveraging the event-driven architecture in Laravel, you can create responsive and efficient systems that handle complex tasks seamlessly. If you’re looking for expert Laravel development services, our team of experienced Laravel developers can help you implement events and listeners in your application to achieve the best results.