How to Send Email Using Laravel Mail Queue?
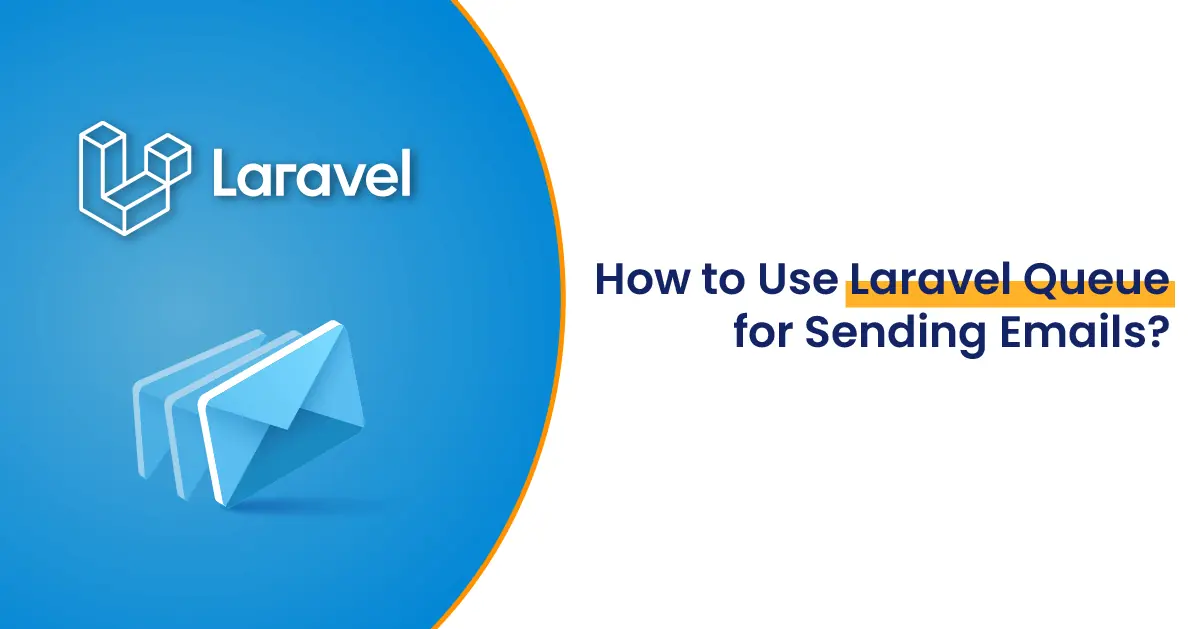
How to Send Email Using Laravel Mail Queue?
Efficient email management is a crucial feature in modern web applications. Whether it’s sending newsletters, notifications, or transactional emails, a seamless email delivery process ensures an enhanced user experience. Laravel, one of the most widely used PHP frameworks, simplifies this process with its robust mail queue system. In this blog, we’ll guide you through the steps to send emails using the Laravel mail queue, an essential skill for any Laravel developer.
Why Use Laravel Mail Queue for Sending Emails?
Emailing is a time-consuming process, especially when dealing with large batches or complex operations. By using Laravel’s mail queue system, you can:
- Improve User Experience: Avoid delays in page loading caused by synchronous email sending.
- Efficient Resource Management: Offload tasks to the background, freeing up server resources.
- Reliability: Handle retries and failed jobs effortlessly.
Step-by-Step Guide to Sending Emails with Laravel Mail Queue
1. Set Up Your Mail Configuration
Start by configuring your .env
file with your mail service credentials:
MAIL_MAILER=smtp
MAIL_HOST=smtp.gmail.com
MAIL_PORT=587
MAIL_USERNAME=your_email@example.com
MAIL_PASSWORD=your_password
MAIL_ENCRYPTION=tls
MAIL_FROM_ADDRESS=your_email@example.com
MAIL_FROM_NAME="Your Application Name"
Test the configuration to ensure your application can send emails.
2. Create a Mailable Class
Laravel makes it easy to define email content using mailable classes. Run the following command:
php artisan make:mail SendEmailTest
This generates a new mailable class in the app/Mail
directory. Update the build
method to include email content:
use Illuminate\Mail\Mailable;
class SendEmailTest extends Mailable
{
public $details;
public function __construct($details)
{
$this->details = $details;
}
public function build()
{
return $this->view('emails.test_email')->with('details', $this->details);
}
}
3. Set Up the Email View
Create an email view file in resources/views/emails/test_email.blade.php
:
<!DOCTYPE html>
<html>
<head>
<title>Test Email</title>
</head>
<body>
<h1>{{ $details['title'] }}</h1>
<p>{{ $details['message'] }}</p>
</body>
</html>
4. Create a Job Class for the Mail Queue
Generate a job class to handle the email-sending logic:
php artisan make:job SendEmailJob
In the SendEmailJob
class, define the handle
method:
use Illuminate\Bus\Queueable;
use Illuminate\Contracts\Queue\ShouldQueue;
use Illuminate\Foundation\Bus\Dispatchable;
use Illuminate\Queue\InteractsWithQueue;
use Illuminate\Queue\SerializesModels;
use Illuminate\Support\Facades\Mail;
use App\Mail\SendEmailTest;
class SendEmailJob implements ShouldQueue
{
use Dispatchable, InteractsWithQueue, Queueable, SerializesModels;
protected $details;
public function __construct($details)
{
$this->details = $details;
}
public function handle()
{
Mail::to($this->details['email'])->send(new SendEmailTest($this->details));
}
}
5. Dispatch the Job from Your Controller
Create or update a controller to dispatch the job:
php artisan make:controller EmailController
In the EmailController
, add the following method:
use App\Jobs\SendEmailJob;
use Illuminate\Http\Request;
class EmailController extends Controller
{
public function sendEmail(Request $request)
{
$details = [
'email' => $request->email,
'title' => $request->title,
'message' => $request->message,
];
SendEmailJob::dispatch($details);
return response()->json(['message' => 'Email sent successfully!']);
}
}
6. Configure the Mail Queue Driver
Laravel supports multiple queue drivers like database, Redis, and SQS. To use the database driver:
- Run the following commands to create the necessary table:
php artisan queue:table
php artisan migrate
- Update your
.env
file:
QUEUE_CONNECTION=database
7. Run the Mail Queue Worker
To start processing the jobs in the queue, run:
php artisan queue:work
Use a process manager like Supervisor to keep the queue worker running in production.
Conclusion
The Laravel mail queue system provides a reliable and efficient way to handle background tasks like sending emails. By following the steps outlined in this guide, you can implement a robust email system that enhances user experience and optimizes resource usage. For businesses seeking professional Laravel development services, partnering with a skilled Laravel developer ensures your application leverages the full potential of Laravel’s features. At OneClick IT Consultancy, we specialize in delivering scalable Laravel solutions tailored to your needs. Contact us today to elevate your application’s performance!