How to Implement Laravel Notification with Pusher for Real-Time Web Updates
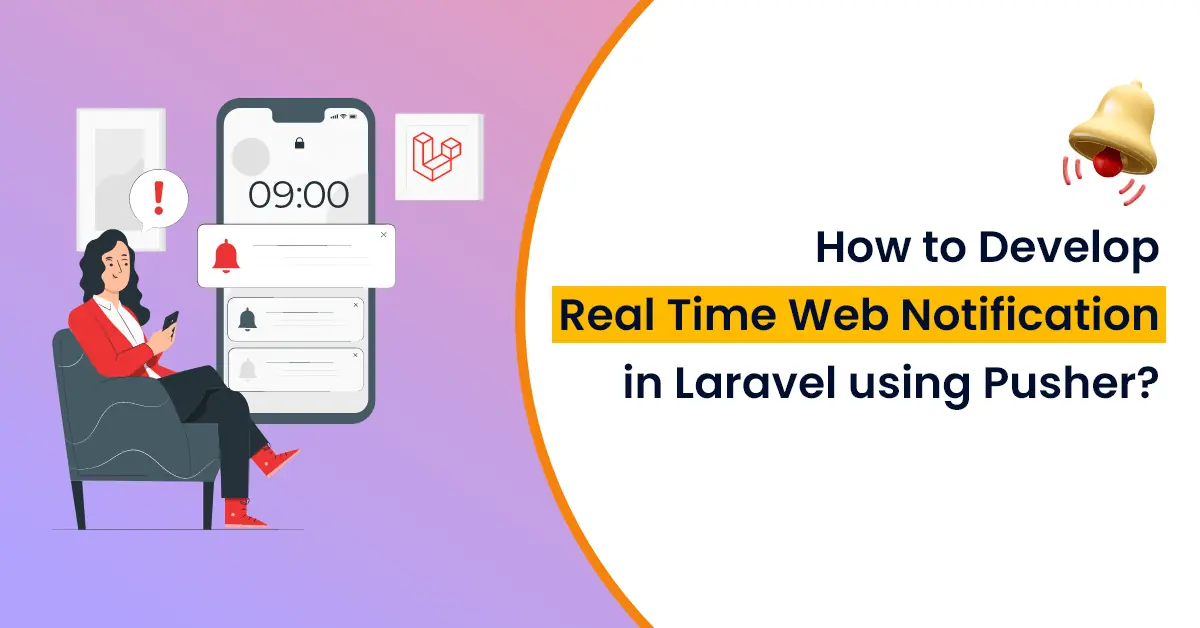
Real-time web notifications have become an essential part of modern web applications. Whether you’re a Laravel developer, building a messaging system, alerting users about new content, or providing real-time updates, the ability to notify users instantly enhances user engagement and experience. In this guide, we’ll walk you through the steps to build a real-time Laravel notification with Pusher, a popular service for handling real-time WebSocket connections.
What is Pusher?
Pusher is a cloud-based service that enables real-time communication in your applications. It uses WebSockets to send messages to clients as soon as an event occurs on the server. Laravel makes it easy to integrate Pusher through its built-in notification system, allowing you to send notifications effortlessly.
Why Use Laravel Notification with Pusher?
Laravel’s notification system simplifies sending notifications to users, whether through email, database, SMS, or even real-time web notifications using Pusher. The integration of notification Pusher Laravel allows you to push notifications to users instantly, providing a seamless experience. You can create a real-time notification Laravel Pusher system for your app without much hassle.
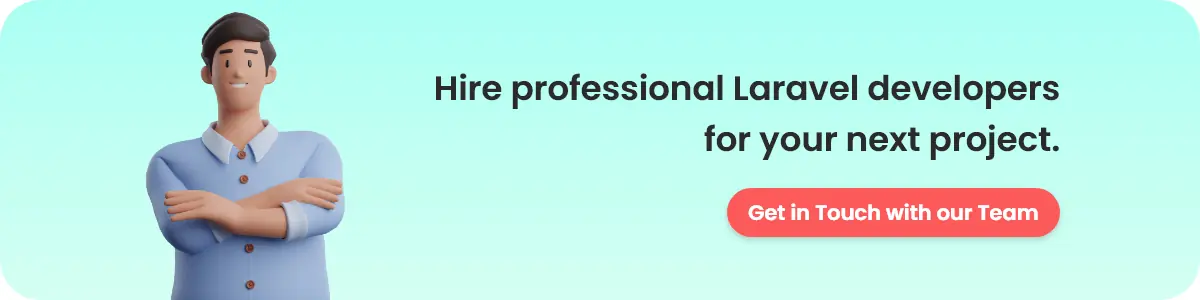
Steps to Implement Real-Time Web Notifications in Laravel with Pusher
Step 1: Install and Set Up Laravel
Start by installing a fresh Laravel project if you haven’t done so already:
bash
composer create-project --prefer-dist laravel/laravel pusher-notifications
Navigate to your Laravel project directory:
bash
cd pusher-notifications
Step 2: Install Pusher and Laravel Echo
Next, you need to install Pusher’s SDK and Laravel Echo. Laravel Echo is a JavaScript library that makes it easy to listen for events broadcast by your Laravel application.
First, install the required packages:
bash
composer require pusher/pusher-php-server npm install --save laravel-echo pusher-js
Read More: Events and Listeners in Laravel
Step 3: Set Up Pusher
Go to Pusher and create an account if you don’t already have one. After logging in, create a new app and note down the credentials like App ID, Key, Secret, and Cluster.
Once you have the credentials, add them to your .env
file:
env
BROADCAST_DRIVER=pusher PUSHER_APP_ID=your-app-id PUSHER_APP_KEY=your-app-key PUSHER_APP_SECRET=your-app-secret PUSHER_APP_CLUSTER=your-app-cluster
Step 4: Configure Laravel Broadcasting
Open config/broadcasting.php
and set the default driver to Pusher:
php
'default' => env('BROADCAST_DRIVER', 'pusher'),
In the same file, ensure the Pusher configuration is correct:
php
'pusher' => [ 'driver' => 'pusher', 'key' => env('PUSHER_APP_KEY'), 'secret' => env('PUSHER_APP_SECRET'), 'app_id' => env('PUSHER_APP_ID'), 'options' => [ 'cluster' => env('PUSHER_APP_CLUSTER'), 'encrypted' => true, ], ],
Step 5: Create a Notification
Now, let’s create a notification that will be sent to the user. Use Laravel’s Artisan command to generate a notification class:
php artisan make:notification RealTimeNotification
In the RealTimeNotification
class, modify the via
method to include broadcast
:
php
public function via($notifiable) { return ['database', 'broadcast']; }
Then, in the toBroadcast
method, define the structure of the notification:
php
public function toBroadcast($notifiable) { return new BroadcastMessage([ 'message' => $this->message, ]); }
Step 6: Broadcasting the Notification
Now, we need to fire the event that will send the notification. In your controller or wherever the action occurs, use the notify
method to trigger the notification:
php
$user->notify(new RealTimeNotification("New message received!"));
Read More: How to Send Email Using Queue in Laravel?
Step 7: Listen for Notifications in JavaScript
On the client-side, we’ll use Laravel Echo to listen for the notification. In your resources/js/bootstrap.js
file, set up the Echo listener:
js
import Echo from "laravel-echo"; import Pusher from "pusher-js"; window.Pusher = Pusher; window.Echo = new Echo({ broadcaster: "pusher", key: "your-pusher-key", cluster: "your-pusher-cluster", forceTLS: true }); Echo.channel("user." + userId) .listen("RealTimeNotification", (event) => { console.log(event); alert(event.message); });
Now, whenever the notification is broadcasted, it will trigger an alert on the client side.
Step 8: Testing the Notification
To test the real-time web notification, fire the notification event through a controller action, and you should see the alert on the frontend whenever the notification is broadcasted.
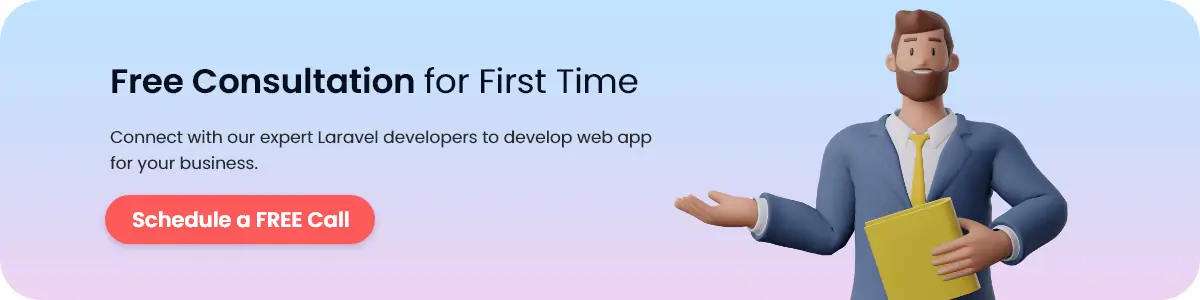
Conclusion
Building real-time notifications in Laravel using Pusher is relatively simple with the help of Laravel’s built-in broadcasting functionality. By following these steps, you can implement a real-time notification system in your Laravel application to enhance user engagement and provide instant feedback.
If you’re looking to integrate real-time notification Laravel Pusher functionality into your application, hiring Laravel developers can help you streamline the process and ensure your app works smoothly. Whether you need custom Laravel development services or a dedicated team to manage your application’s features, our expert developers at OneClick IT Consultancy can assist you in building top-notch, real-time solutions.