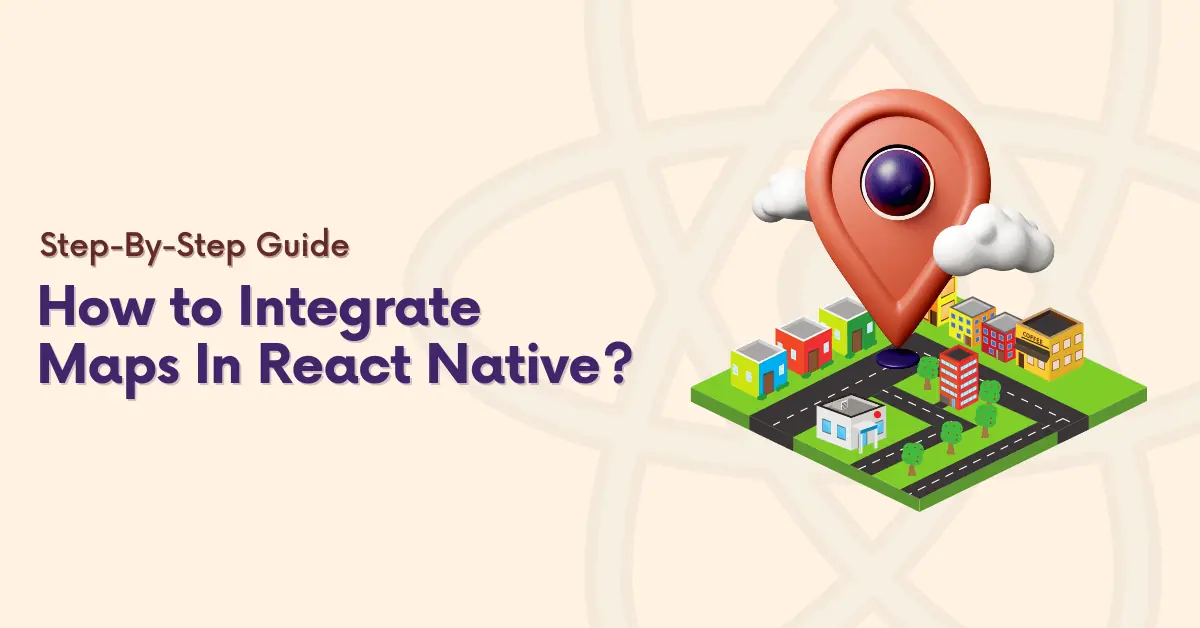
Introduction of React Native Map
React Native Maps is a powerful library widely used and maintained for adding map functionality to iOS and Android applications. React Native development allows you to integrate rich map features, such as navigation routes and arrival directions within the app. By using the MapView
component and Marker
, developers can easily embed Google Maps or other map services, allowing for interactive location-based features like setting pins and placing icons in specific locations.
In this guide, we’ll walk you through the step-by-step process of integrating React Native Maps into your application for both Android and iOS. Let’s start enhancing your app’s functionality with seamless map integration!
Statistics of React Native Map Integration:
- React Native maps library has more than 96,445 weekly downloads.
- 4.6 rating out of 5 of this library.
- 1,10,000 users.
- 400 contributors.
Component of React Native Map Integration
- <Marker/>
- <Mapview/>
- <Polygon/>
- <Overlay/>
- <Heatmap/>
- <Geojson/>
- <Callout/>
- <Polyline/>
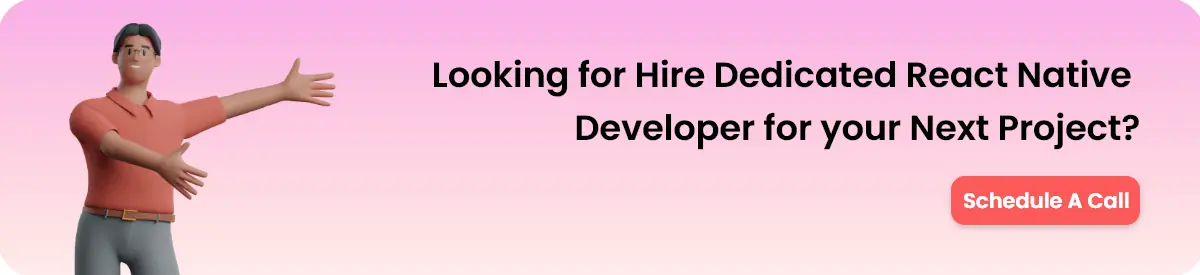
Standard Process of React Native Map Integration:
Below are the 10 steps to integrate maps into your Android and iOS devices.
1. Add Map dependencies in your package.json file:
“react-native”:”0.64.2”.
“react-native-maps”: “0.28.0”.
2. Install the React Native Map package:
Run npm i react-native-maps in the terminal after creating the project.
3. Configuration on iOS:
Run react-native link after react-native-maps in the terminal.
After this you should be able to use this library.
4. POD Files changes:
pod 'GoogleMaps'
Pod ‘Google-map-ios-utils’’
pod 'react-native-maps', path: '.././..’
5. Create a Google Map API Key:
- Using Google Map Platform generates an API key.
6. Steps to enable the API_KEY:
- Create new or add your project
- Go to credentials.
- Add credentials to your added project
- Click on Google Maps SDK for iOS.
- Enable Google Maps SDK.
- Click on Continue of the generated Popup. After the above steps, we will get the API key. Copy that API key and add it into the APPDelegate.m file.
#import <GoogleMaps/GoogleMaps.h>
[GMSServices provideAPIKey:@’’Google_API_Key”]
7. Import React Native Map into App.js:
import MapView from react-native-maps
8. Creating the map into view:
<View>
<MapView
style={styles.map}
initialRegion={{
latitude: 37.78825,
longitude: -122.4324,
latitudeDelta: 0.0922,
longitudeDelta: 0.0421,
}}
/>
</View>
9. App.js file:
import React, { Component } from 'react';
import MapView ,{ PROVIDER_GOOGLE } from react-native-maps;
Const App=()=>(
{
return (
<View>
<MapView
provider={PROVIDER_GOOGLE}
style={{flex:1}}
initialRegion={{
latitude: 37.78825,
longitude: -122.4324,
latitudeDelta: 0.0922,
longitudeDelta: 0.0421,
}}
/>
</View>
)}
Export default App;
10. Run on your iOS device:
Run react-native-iOS into the terminal and open the simulator to view output.
Benefits of React Native Map
React Native Map library makes it easy to integrate maps. Using , you can implement different features on Android and iOS. It has many options like adding markers, adding extra API components, track particular location, programmatically changing location, zoom in-out particular locations.
Mapview animated to specified region and location. Few things are available only in Android and few things are available in iOS.
Conclusion
React Native Maps provides a powerful solution for embedding maps within both Android and iOS applications. By integrating maps into your React Native apps, you can enhance user experiences in on-demand applications like delivery, transportation services, and more. This functionality not only boosts engagement but also offers users real-time location insights, adding significant value to your app.
Ready to transform your app’s capabilities? Hire React Native developers today to unlock the full potential of React Native Maps and deliver an engaging, location-based experience for your users!
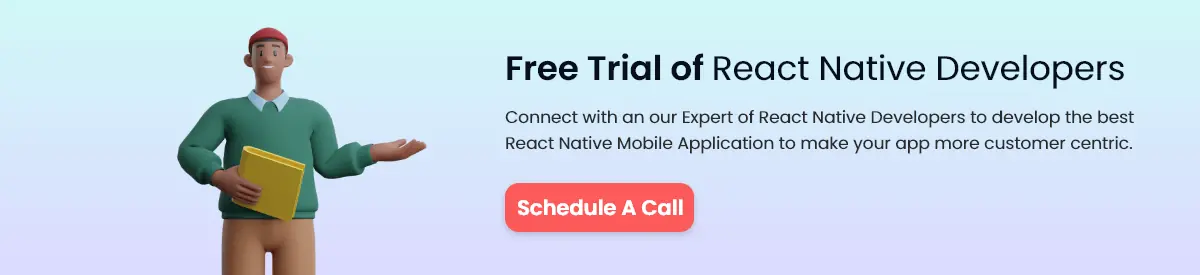
FAQs
In the Mapview tag, Set the region of user location and declare shows UserLocation true.
Latitude: North-to-south distances are displayed on the map.
Longitude: East-to-west distances are displayed on the map.
The map is styled by React native according to the width and height.