PayPal Express Checkout Integration with Laravel 5
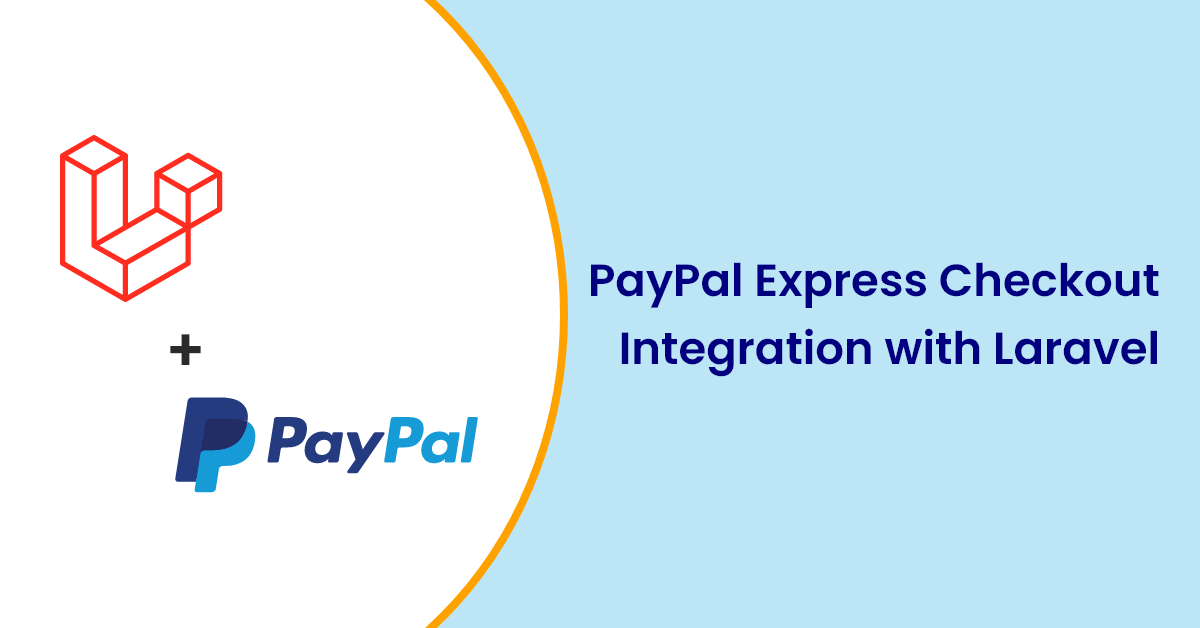
What is PayPal?
PayPal is a globally recognized company that operates an online payment system supporting worldwide money transfers. It serves as an electronic alternative to traditional money exchange systems. PayPal acts as a payment gateway for online sellers, auction sites, and many other commercial entities, charging a fee in exchange for its services. By utilizing PayPal express checkout Laravel integration, developers can seamlessly add secure and efficient payment processing to Laravel applications, ensuring a smooth transaction experience for both buyers and sellers. This payment system is commonly referred to as PayPal.
In this blog, we will check and focus on how to get paypal express checkout Laravel integration to make it easier for Laravel developers.
What is a Payment Gateway?
A payment gateway is a merchant service provided by any e-commerce application service provider that authorizes the processing of credit card or direct payments for online businesses, retailers, or traditional brick-and-mortar stores. It facilitates payment transactions by transferring information between a payment portal (such as a website or mobile app) and the front-end processor or acquiring bank.
Configuring PayPal Express Laravel Integration with Your App
To integrate PayPal Express Checkout Laravel, we need to first install the PayPal SDK using Composer.
bash
composer require paypal/rest-api-sdk-php
Next, log into PayPal’s developer mode, create a new sandbox account, and retrieve the keys (client_id and secret) for testing this PayPal Express Checkout Laravel integration.
- Visit developer.paypal.com to create a merchant account.
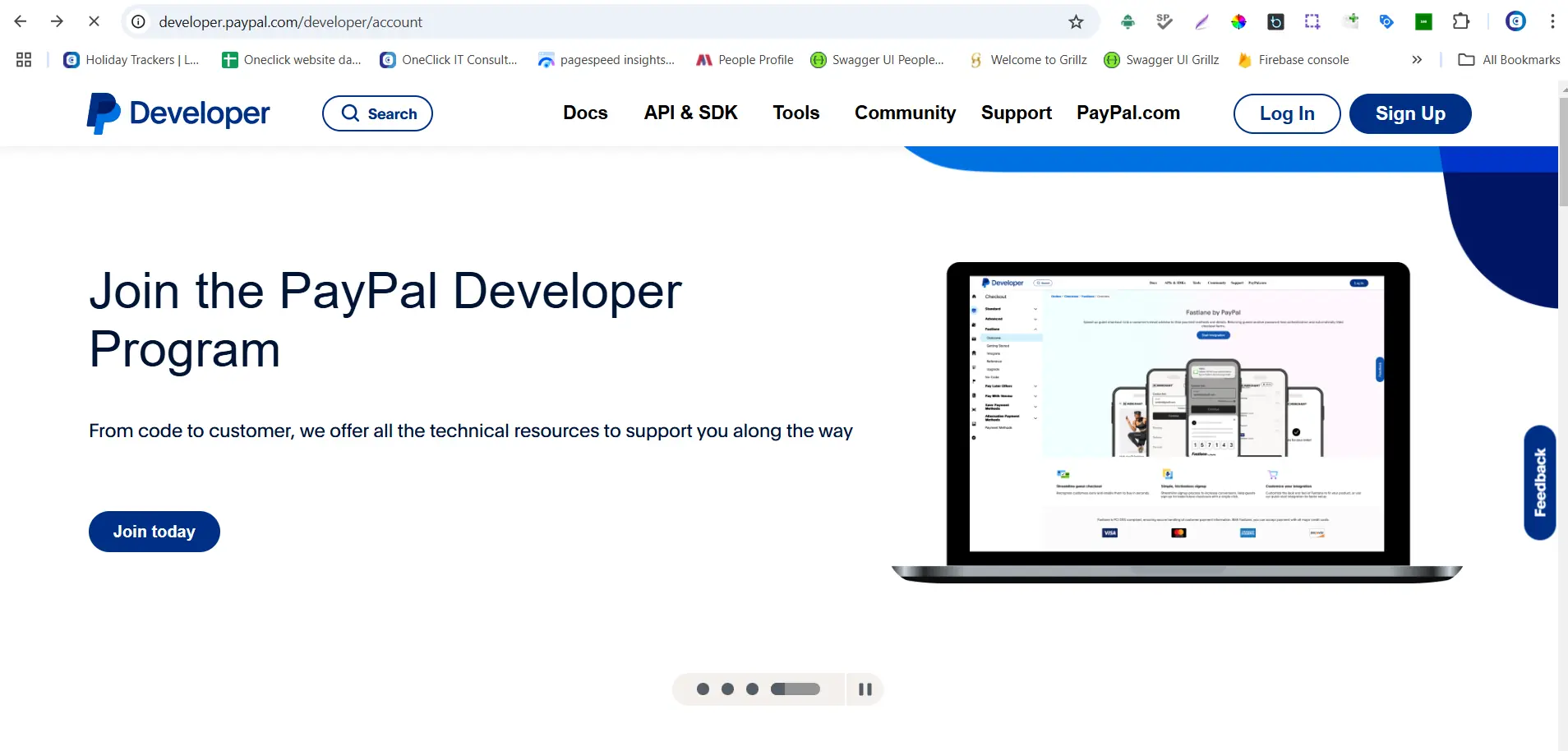
- Create both merchant and buyer test accounts to simulate transactions.
- Generate a new app to get the client_id and secret keys.
Once your app is created, copy the CLIENT_ID
and SECRET
keys and paste them into your .env
file for secure storage.
PAYPAL_CLIENT_ID=
PAYPAL_SECRET=
PAYPAL_MODE=sandbox
Now, create a new file paypal.php
in the config
directory and add the following configuration:
<?php
return [
'client_id' => env('PAYPAL_CLIENT_ID', ''),
'secret' => env('PAYPAL_SECRET', ''),
'settings' => [
'mode' => env('PAYPAL_MODE', 'sandbox'),
'http.ConnectionTimeOut' => 30,
'log.LogEnabled' => true,
'log.FileName' => storage_path() . '/logs/paypal.log',
'log.LogLevel' => 'ERROR'
],
];
PayPal Express Laravel Payment Form
To start accepting payments via PayPal, add a payment form to your website. Below is a simple form to collect an amount and initiate PayPal Express Checkout Laravel.
html
<form class="w3-container w3-display-middle w3-card-4" method="POST" ids="payment-forms" action="/payment/add-funds/paypal">
{{ csrf_field() }}
<h2 class="w3-text-blue">Payment Form</h2>
<p>Demo PayPal form - Integrating PayPal in Laravel</p>
<p>
<label class="w3-text-blue"><b>Enter Amount</b></label>
<input class="w3-input w3-border" name="amount" type="text">
</p>
<button class="w3-btn w3-blue">Pay with PayPal</button>
</form>
PayPal Express Checkout Payment Controller
Next, create a controller to handle PayPal Express Checkout Laravel Integration:
bashCopy codephp artisan make:controller PaymentController
In the constructor of the controller, initialize PayPal configuration and API context.
php
public function __construct()
{
$paypal_conf = \Config::get('paypal');
$this->_api_context = new ApiContext(new OAuthTokenCredential(
$paypal_conf['client_id'],
$paypal_conf['secret']
));
$this->_api_context->setConfig($paypal_conf['settings']);
}
Handling the Payment
After the user enters an amount and clicks the “Pay with PayPal” button, the following function executes.
php
public function payWithpaypal(Request $request)
{
$payer = new Payer();
$payer->setPaymentMethod('paypal');
$item_1 = new Item();
$item_1->setName('Item 1')
->setCurrency('USD')
->setQuantity(1)
->setPrice($request->get('amount'));
$item_list = new ItemList();
$item_list->setItems([$item_1]);
$amount = new Amount();
$amount->setCurrency('USD')
->setTotal($request->get('amount'));
$transaction = new Transaction();
$transaction->setAmount($amount)
->setItemList($item_list)
->setDescription('Your transaction description');
$redirect_urls = new RedirectUrls();
$redirect_urls->setReturnUrl(URL::route('status'))
->setCancelUrl(URL::route('status'));
$payment = new Payment();
$payment->setIntent('Sale')
->setPayer($payer)
->setRedirectUrls($redirect_urls)
->setTransactions([$transaction]);
try {
$payment->create($this->_api_context);
} catch (\PayPal\Exception\PPConnectionException $ex) {
\Session::put('error', 'Connection timeout');
return Redirect::route('paywithpaypal');
}
foreach ($payment->getLinks() as $link) {
if ($link->getRel() == 'approval_url') {
$redirect_url = $link->getHref();
break;
}
}
Session::put('paypal_payment_id', $payment->getId());
if (isset($redirect_url)) {
return Redirect::away($redirect_url);
}
Session::put('error', 'Unknown error occurred');
return Redirect::route('paywithpaypal');
}
PayPal Payment Status
After processing the payment, verify the payment status and display success or failure messages accordingly.
php
public function getPaymentstatus()
{
$payment_id = Session::get('paypal_payment_id');
Session::forget('paypal_payment_id');
if (empty(Input::get('PayerID')) || empty(Input::get('token'))) {
\Session::put('error', 'Payment failed');
return Redirect::route('/');
}
$payment = Payment::get($payment_id, $this->_api_context);
$execution = new PaymentExecution();
$execution->setPayerId(Input::get('PayerID'));
$result = $payment->execute($execution, $this->_api_context);
if ($result->getState() == 'approved') {
\Session::put('success', 'Payment successful');
return Redirect::route('/');
}
\Session::put('error', 'Payment failed');
return Redirect::route('/');
}
Conclusion
Integrating PayPal Express Checkout in Laravel can significantly improve your users’ online payment experience. Following the steps outlined above, you can easily set up a PayPal Express Checkout Laravel integration.
Contact us today if you need further assistance with PayPal Express Checkout in Laravel integration or require custom Laravel development services. Our team of skilled Laravel developers can help you implement a seamless payment system for your Laravel application.