SwiftUI Importants: Best Practices for Developers
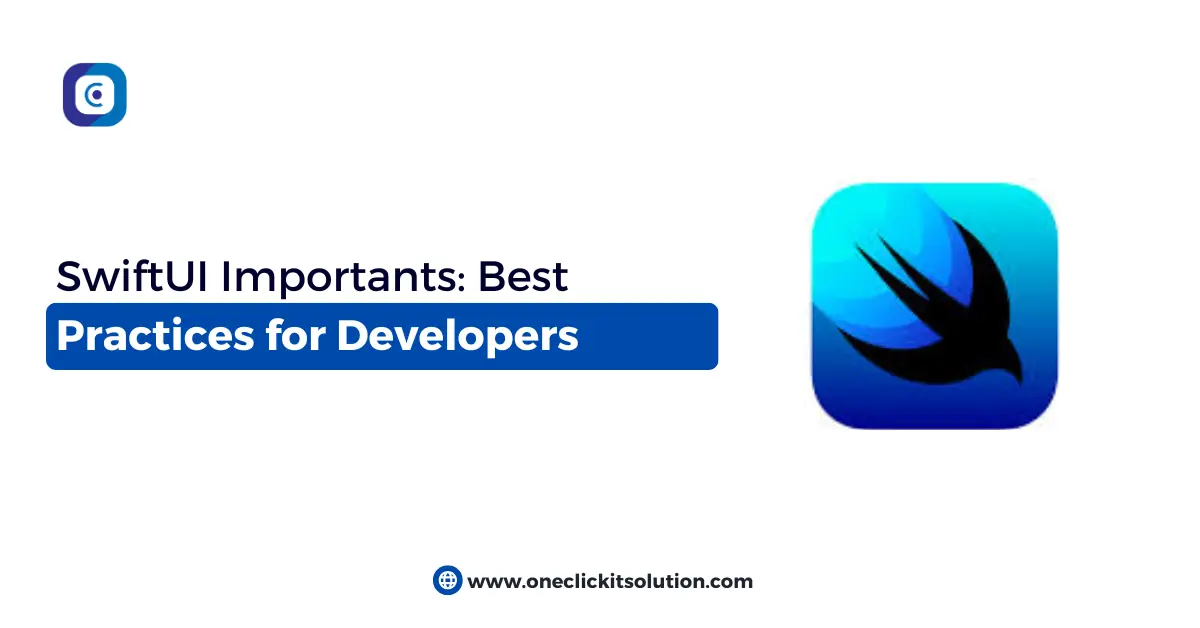
Diving deep into SwiftUI This blog post drops us into SwiftUI: the modern, developer-friendly framework to create gorgeous user interfaces in iOS, iPadOS, macOS, watchOS, and tvOS. We will cover essential best practices for writing clean, maintainable, and performant SwiftUI code, pointing out why SwiftUI stands out from UIKit and cross-platform solutions.
Introduction to SwiftUI
SwiftUI is a declarative UI framework of iOS development, in which you write what the desired state of the UI is, rather than specifying how to build it step by step. This creates code that is short and readable, thus allowing you to have a clear view of the structure of the applications you are setting up. Other than that, SwiftUI will really integrate with the latest Apple technologies and you will be able to build your apps smoothly across all of your platforms.
Mastering SwiftUI Best Practices
Embrace Declarative Syntax:
No more imperative code! You can declare both UI elements and their properties, which means much cleaner and readable code. Here’s an exam
Import SwiftUI
struct ContentView: View < var body: some View <
VStack <
Text("Welcome to SwiftUI!")
font(.largeTitle)
foregroundColor(.blue)
Button(action: {
print("Button tapped" )
) {
Text ("Tap me")
Manage State Effectively:
There are several property wrappers proposed by SwiftUI for state management. These fall into three main kinds, and so on as follows:
@State: Manages local state within a view.
@Binding: Enables two-way data flow between parent and child views.
@Environment: Accesses global application state.
Proper usage of these wrappers helps keep your UI in step with changes in the underlying data.
Build Reusable Components:
Apply the DRY-Don’t Repeat Yourself principle as applicable in SwiftUI; make a set of custom views for reuse of common-used UI, cutting off duplication, and making code easier to maintain.
import SwiftuI
struct CustomButton: View <
let title: String let action: () -> Void
var body: some View <
Button(action: action) <
Text(title)
padding)
background(Color.blue)
foregroundColor(-white)
.cornerRadius (8)
Leverage Navigation and Transitions:
Providing built-in navigation tools, such as NavigationLink and a smooth transition for a great UX, SwiftUI makes it easy to avoid managing your controllers and keep your navigation stack clean.
Optimizing Performance in SwiftUI
Lazy Loading for Large Datasets:
LazyVStack or LazyHGrid must be used if you have a lot of items in a list or grid to ensure lazy loading without performance bottlenecks. These views only load the items as you scroll and are becoming visible on the screen.
struct CustomList: View < let title: String let action: () →> Void
var body: some View <
LazyVStack {
ForEach(0..<1000) { index in
Text("Item \(index)")
Separate Concerns with MVVM:
The Model-View-ViewModel pattern really promotes clean separation of code. You would use @ObservedObject to wire a view up with its ViewModel, the layer which encapsulates the data and business logic, and therefore keeps your UI code tightly focused on presentation.
class CounterViewModel: ObservableObject <
@Published var count = 0
func incremento <
count += 1
struct CounterView: View <
@ObservedObject var viewModel = CounterViewModel()
var body: some View t
VStack (
Text( "Count: \(viewModel.count)")
Button ("Increment") < viewModel. increment)
Cross-Platform Benefits of SwiftUI
The best UI development for the whole Apple ecosystem is on the banner of SwiftUI. You can write the UI for iOS, iPadOS, macOS, watchOS, and tvOS on a single codebase and save your time and efforts when implementing the same UI on multiple platforms.
Diefference: SwiftUI vs. UIKit
- Fewer Boilerplate: SwiftUI uses fewer lines than UIKit so a lot faster to develop
- Live Previews: SwiftUI provides real-time previewing of UI that allows for quick iteration and design without even running the simulator.
- Declarative vs. Imperative: The former has to do with what it is to want, while the latter has to do with how you achieve it.
- Learning Curve: SwiftUI is easier to learn because it is declarative.
- Interoperability: UIKit is more interoperable with existing iOS code.
- Performance: Of course, UIKit was always the fastest historically, but SwiftUI is not bad itself.
- Development Speed: SwiftUI is faster as it uses a declarative syntax.
- Cross-Platform: SwiftUI can be used on various platforms, including iOS UIKit.
Advantages and Disadvantages of Swift and SwiftUI.
Swift and SwiftUI provide fast development, modern syntax, and cross-platform compatibility but come with a steep learning curve and limited backward compatibility.
Pros of Using Swift for iOS Development
Swift was invented by Apple in the year 2014, and these new features are actually embedded to replace Objective-C. It is used for developing applications related to iOS, macOS, watchOS, and tvOS in the modern world. Following are some of the most notable benefits that make it a very powerful language in the development world.
Performance and Speed:
Swift aims to be high-performance, which quite often can match C++ speed for many types of tasks. It helps greatly especially in applications where speed becomes a major issue because of resource intensity.
Type Safety and Error Handling:
The language has a strong type system, which automatically removes bugs and makes the code more dependable. Optional types help manage null or missing data safely, and the try-catch error handling system adds one more layer of dependability.
Concise Syntax:
Swift has a clean modern syntax that makes code readable and writable. This eliminates much of boilerplate code thus letting developers be more productive.
Cross-Platform Potential:
Swift’s open-source nature allows it to be adopted for server-side development with frameworks like Vapor and Kitura. Applications are no longer limited to the Apple’s ecosystem.
As Active Community and Tooling Support:
Swift is supported by Apple, along with the community that’s actively working towards its new feature addition and improvements. The tailored tools at an ecosystem of Apple, particularly Xcode make the hassle-free development.
Memory Management:
ARC in Swift allows automatic memory management where the existence of unused objects is tracked and eliminated to reduce memory usage without needing any developer intervention.
Pros of SwiftUI in Production
Declarative Syntax:
With SwiftUI, you can actually tell it how the UI should look in terms of its current state, and the code becomes more readable and more maintainable. It means automatically handling updates by itself.
In general, the code is shorter and cleaner than the UIKit’s code, which makes development faster. This is because, in Xcode, its real-time preview allows for quicker feedback without needing repeated builds and runs of the application to try different implementations.
Cross-Platform Support:
The components in SwiftUI have been built to work across iOS, macOS, watchOS, and tvOS with minimal changes between each, which can help in developing multi-platform applications based on shared code.
Fast updates with reactive UI:
Using @State, @Binding, and @ObservedObject state management APIs in SwiftUI is ideal for modern, data-driven applications because it enables dynamic, reactive UI updates.
Interoperability with Combine:
SwiftUI uses the Apple’s combine framework, which manages the flow of data asynchronously in order to handle streams of data, networking, or even user-driven events.
Cons of SwiftUI in Production
Not fully functional API and Customization:
SwiftUI is very powerful, but it’s also missing some more advanced customization options and pieces that UIKit has. For example, parts of the UI, maybe working with complex animations or requiring stricter control over elements, may better be implemented with UIKit.
Changeable Framework:
SwiftUI is still under growth, as new components along with new features are coming up every year. This attribute of growth symbolizes that the code written for one version may need to be refactored in the next release versions of SwiftUI.
Advanced Features Require a Steep Learning:
Curve The basics of SwiftUI are accessible, but the features that might use custom gestures, animations, or Combine integration require a more thorough understanding of both Swift and functional programming concepts, which are time-consuming to learn.
Debugging Complexity:
It’s pretty hard to debug more complex code in SwiftUI, especially with advanced bindings and state management. The error messages can be kind of cryptic, and it’s not as simple sometimes to track the bugs through the declarative syntax as it is with implementing in UIKit.
Look Into Some Popular Swift Packages to Improve Your iOS App:
Swift Package Manager (SPM) has made it easier for us to add third-party libraries to your Swift projects. Here are some of the most popular and versatile packages for enhancing your iOS applications:
Alamofire: Networking
Alamofire: is a swift networking library, powerful and elegant, simplifying even making HTTP requests, handing JSONs, or managing network sessions. Such features like request retrying, caching, and integration with Codable make Alamofire the go-to choice for developers who need a strong networking solution.
SwiftyJSON Use for: JSON Parsing
Although SwiftyJSON makes the process of JSON parsing easier with Swift’s Codable, SwiftyJSON is still more preferred due to its flexibilities and easier usage, especially in JSON structures complexities. It’s also very helpful for projects having dynamic JSON parsing or using the data in the nested structures.
Kingfisher Usage: Image Caching
It is an efficient and user-friendly library when it comes to downloading and caching images. It even supports on-disk and in-memory caching, animated GIFs, and progressive image loading. This package is pretty suitable for applications that display remote images in a performant way.
SnapKit Used for: Auto Layout
SnapKit SnapKit makes working with Auto Layout constraints much easier, thanks to a clean DSL-based syntax that lets you compose complex layouts programmatically. It is really useful when you are developing adaptive layouts that need to work on multiple screen sizes without the hassle of writing cumbersome constraint code.
Firebase: Use for Analytics, Database, Authentication and a lot more
In terms of features, Firebase is a complete backend solution that includes the following: real-time database, analytics, user authentication, cloud storage, and push notifications. It gives tools that can handle backend services without having to set up your own server, making it suitable for small to medium-sized apps.
Highest-Scored Networking, Persistence, and UI Enhancement Libraries in Swift
Networking Libraries: Moya
Moya is a network abstraction layer built on top of Alamofire. This means it’s so much easier to actually define API endpoints with a cleaner, modular approach much better approach for large codebases and lots of endpoints.
Combine
Combine, by Apple, is a reactive framework that works pretty seamlessly for handling asynchronous data when you are using SwiftUI. It best suits the management of API responses, user input, and data streams if apps are mainly built on SwiftUI or extensive reactive programming.
URLSession
URLSession, even though this is part of Swift, can also be further extended by adding custom extensions for more robust error handling and retries of the request along with network tracking. Combine URLSession with Alamofire and Combine to fill nearly any networking need.
Persistence Libraries
Core Data
Apple’s Core Data: This is one of the fantastic frameworks for managing model objects inside a persistent storage. Despite the significant curve to learn, Core Data is so good for applications that require any sort of complex data models, querying data, or versioned storage.
UserDefaults
UserDefaults is a lightweight persistence tool good for the storage of small amounts of data or user settings. If combined with libraries like Defaults, a Swift package that extends UserDefaults, it is good for simple key-value storage needs.
GRDB
GRDB is actually an SQLite library for Swift. It covers advanced features like full-text search and custom migrations. What fits very well in the need of every application based on structured storage which is familiar and uses SQL but has the additional advantage of using Swift’s type-safe model definitions.
UI Enhancement Libraries
Lottie
Lottie is acknowledged as one of the leading animation libraries that a developer can use to add animations to an app. Lottie enables developers to use JSON-based animations created in Adobe After Effects and makes it pretty easy to add rich, interactive animations with a minimum amount of code.
Hero
Hero is a very powerful library designed to help developers make custom view transitions. Being declarative in syntax, Hero is advisable for developers who have complex animations between views. This is perfect for giving an iOS app dynamic transitions and interesting UI effects.
SkeletonView
SkeletonView is a library to animate placeholder loading states to be more realistic. It engages the user by displaying animation while the content is being loaded smoothly in the background, giving the perceived performance improvement of the application.
Charts by Daniel Gindi
Charts is a library of creating charts to visualize the data. This is then used in apps that require the visualization of big and complex data. Therefore, it is possible to create various kinds of customizable charts, including line, bar, pie, and scatter plots, which are enabled by using charts. Charts is widely employed in apps that require complicated data to be displayed to the user: fitness trackers and financial apps, for example.
SwiftUI – Introspect
SwiftUI-Introspect is basically a bridge that combines two of these technologies to let developers access UIKit properties and methods within SwiftUI views. It really comes in handy when you wanSwiftUI-Introspect is basically a bridge that combines two of these technologies to let developers access UIKit properties and methods within SwiftUI views. It really comes in handy when you want to customize certain SwiftUI components in ways that aren’t natively supported.
Final Word
SwiftUI provides a powerful and efficient way to build user interfaces across Apple platforms with its declarative syntax and state management features. By following best practices such as leveraging reusable views, handling state effectively, and optimizing performance, developers can create intuitive, maintainable, and responsive applications. SwiftUI’s integration with the Swift language and Apple ecosystems makes it a key tool for modern app development. Choose SwiftUI for new projects and cross-platform development. Consider UIKit for existing iOS codebases or performance-critical apps.
FAQs:
Which framework is better for beginners?
Generally, SwiftUI is less complex than UIKit, which is evident for beginners because it is based on the declarative programming paradigm and has fewer lines of code.
Can I use SwiftUI and UIKit together in the same project?
Yes, you can mix SwiftUI and UIKit components in the same project. However, for a more cohesive approach, it’s generally recommended that new projects with SwiftUI be started.
Which framework is better for complex layouts and animations?
Both SwiftUI and UIKit can handle complex layouts and animations. SwiftUI’s declarative syntax can make it easier to manage complex layouts, while UIKit offers more granular control over animations.
Is SwiftUI suitable for large-scale production apps?
Yes, SwiftUI is suitable for large-scale production apps. It has matured significantly, and many successful apps are built using SwiftUI.